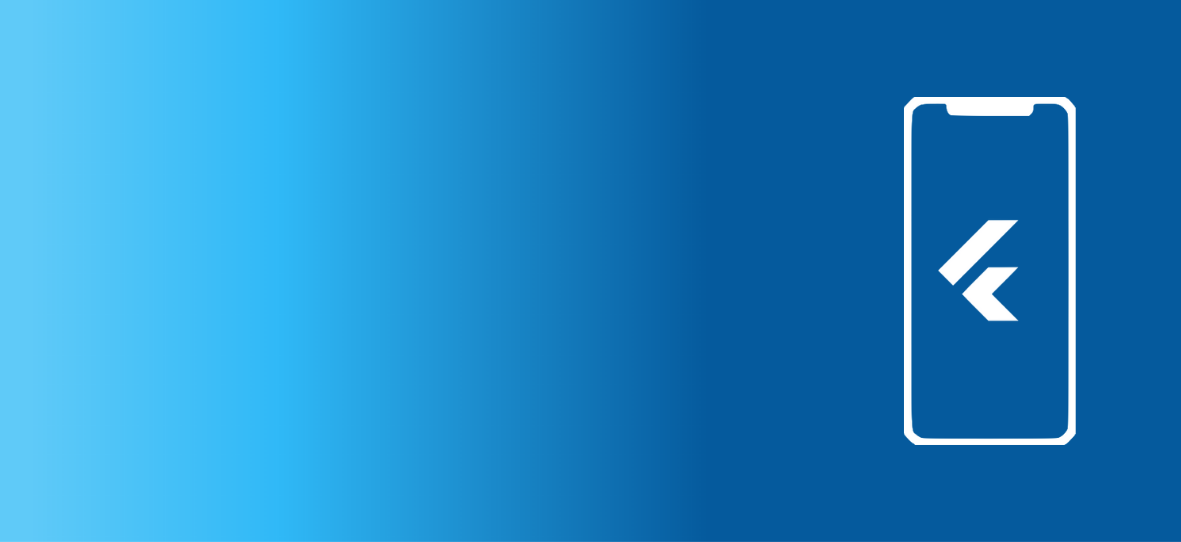
Internationalization in Flutter
The internationalization libraries we considered while building an app with Flutter.
The internationalization libraries we considered while building an app with Flutter.
When you are building an app, you have almost always to take care of internationalization. It is also a good practice to have all string resources stored in one place instead of hardcoding them within the code of your app.
Let’s start with Flutter “out of the box solution.” First of all, you get the internationalization package that lets you make your app shine in different languages across the devices. It also gives you a possibility to extract your string resources to external *.arb file.
At this point, what you have to do is to provide context when extracting string resources. Dear Android Developers…does this sound familiar?
Now you need to create a file with bindings between your app and those generated *.arb files. If you do your development using Android Studio, there is a simple solution for it. It is called Flutter-i18n plugin. Be sure this one will do the job right.
What’s the catch then? There is no catch. We even applied this solution to another app, and it worked perfectly fine! Later on, we started thinking of a possibility to make it even simpler. This process eventually brought us to the idea of creating a straightforward solution for cases in which there is no need for using advanced options.
While working on one of our apps, we were keen to get an iOS-like solution that gives access to the string without passing any context or similar stuff.
What inspired us firmly was the NSLocalizedString class. We did not need to use complexed stuff such as plurals, descriptions, and other components offered by a standard solution. So, we created a library that loads *.json file with translation during the app startup. You can find it here.
Quite frankly, if you do not want to pass context everywhere and use simple localization, this is an excellent fit for you.
The configuration is pretty painless and straightforward. What do you need to do that makes it different from the other libraries? You get to wrap your main widget with “`LocalizedWidget “`, prepare .json files with naming pattern – language.json, e.g., en.json, de.json, pl.json, etc. Then put them into the assets folder.
Remember to set up pubspec.yaml file:
flutter_localizations:
sdk: flutter
flutter_localized_strings: 0.0.3
then you have to add within `flutter:` section:
assets:
- assets/
Modify your “`main.dart“` file:
@override
Widget build(BuildContext context) {
return MaterialApp(
localizationsDelegates: [
// ... app-specific localization delegate[s] here
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
],
supportedLocales: [
const Locale('en', 'US'), // English
// ... other locales the app supports
],
title: "title",
theme: ThemeData(
primarySwatch: Colors.blue,
), home: LocalizedWidget(widget: MyHomePage()),
);
}
Once you have done all of these steps, you should be able to use the library as follows:
LocalizedString.by(key: "title")
while `en.json` looks like this:
{
"title" : "Main title"
}
Remember to add proper imports, though.
Wrapping up, if you wonder which library would be the best one to use, there is no straightforward answer. In case you want a simple solution without creating any additional classes for localization, the “`flutter_localized_string“` seems to be the right choice. If you want more advanced functions and options such as plurals or placeholders, the “out of the box” solution is a better choice for sure.
That’s it. I hope you will find it useful and get to create beautiful internationalized apps no matter what tool you finally choose.
Do you have any questions about our work with Flutter, related projects, or would you like to share your feedback? Let’s get in touch.
Are you looking for a tech partner? Searching for a new job? Or do you simply have any feedback that you'd like to share with our team? Whatever brings you to us, we'll do our best to help you. Don't hesitate and drop us a message!
Drop a message