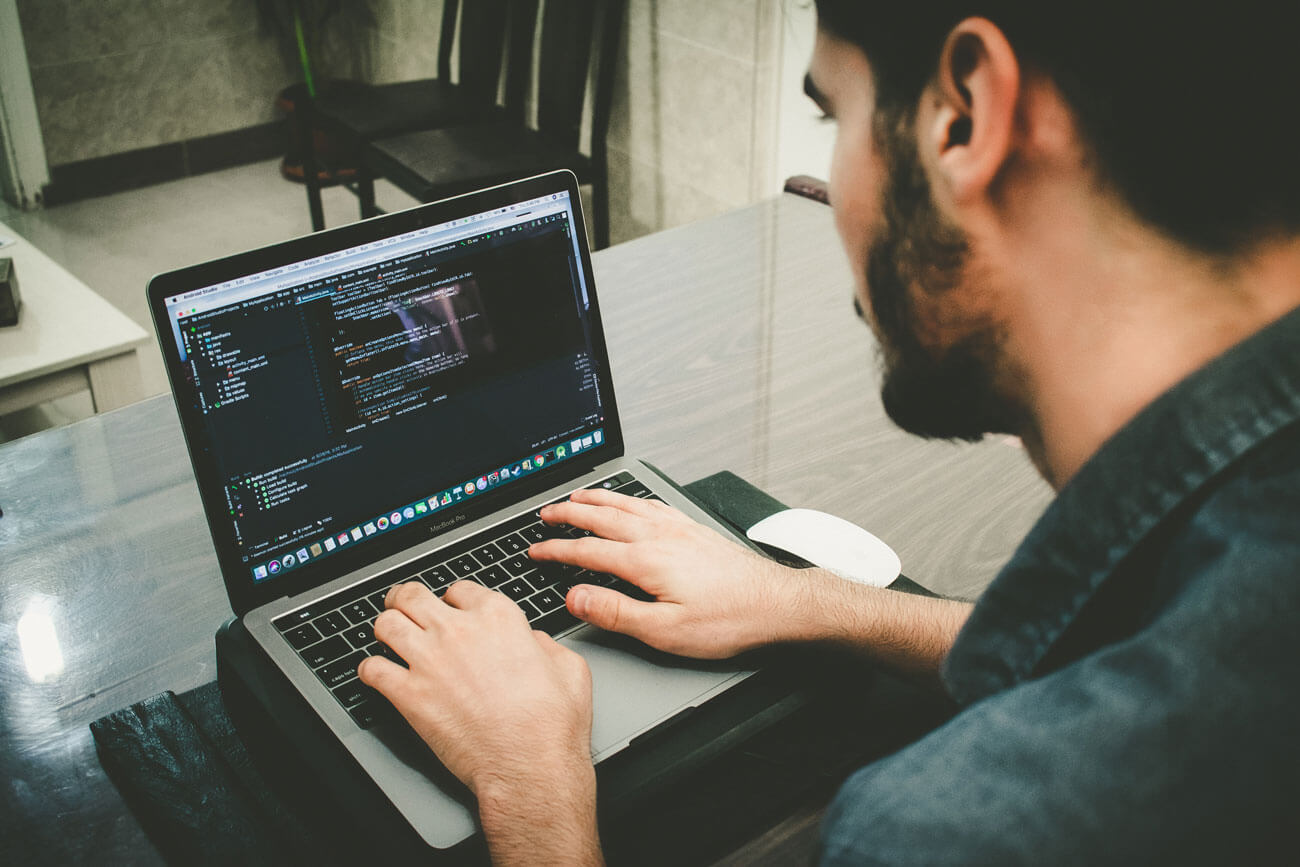
How to Build a Progressive Web App
We focus on the essentials of PWA and walk you through our demo, so you can make your cross-platform application step by step.
We focus on the essentials of PWA and walk you through our demo, so you can make your cross-platform application step by step.
Many popular tools we use every day are progressive web apps. You may not know it — and that’s the point. Uber, for example, launched a PWA in 2017 to make it easier for riders to use the app in poor mobile network conditions or for people to just hail a ride without downloading the native app. Tinder also created a PWA so people who want to just try the dating app can explore the service casually without having to commit to installing it. Very on-brand for Tinder, after all.
Just what a progressive web app is — and how they differ from standard web pages — is what makes these apps so interesting and lucrative for businesses.
At their core, PWAs are types of software that you access over the web. They work across platforms and devices and don’t require separate code bases for different operating systems. This offers better engagement and can greatly increase the amount of time users spend interacting with an app.
PWAs emphasize intuitive user experiences and have largely emerged out of market demands for usable, responsive tools. They’re part of a larger trend in software development that highlights utility regardless of platform, device or network connection.
If you want your business to stay relevant, or to gain an advantage over competitors, responding to the needs of the market is key to success and growth. PWAs have opened services like Uber and Tinder to users who may not have used them otherwise and have led to better usability, more ad revenue and more sales.
Progressive web apps work on web-standard compliant browsers and make it easier for developers to build cross-platform applications. Rather than build a native app for each platform and operating system, developers can build a PWA that runs through standard browsers instead.
To be a PWA, the app should work for users regardless of browser choice or device. In this way, they’re both progressive and responsive. Essentially, users should get an optimal experience whether they’re on a desktop, tablet or mobile device. PWAs rely heavily on vector graphics that scale up or down depending on screen size. These help the apps reduce throughput, making them faster. These apps also account for future forms that have yet to appear. So if you develop a PWA for the current market, it should still work when new technologies emerge.
In terms of usability, PWAs run faster after they’ve loaded initially. Content you see at first stays current and doesn’t have to reload at each session. By the same token, once the app has loaded, it will still work if you lose internet connection or you’re working on a poor-quality network. Service workers, a key part of PWAs, enable the app to collect requests from the main server and then update content once you’ve regained a stable network connection. Service workers also keep the app up to date.
These key features allow your app to be “offline first.” If you have no internet connection, your PWA weather app, for example, can access cached data and retain some functionality. It will update once you regain connection, but in the meantime, you’ll still be able to check the forecast.
Additionally, navigation on a PWA has to feel app-like. So for example, you may get push notifications and other interactions that standard web pages lack. These interactions are part of what make PWAs more engaging and keep users on the platform longer to increase conversions or to improve usability.
Security and shareability are key criteria for progressive apps as well. You have to serve the PWA through HTTPS to ensure that no one has tampered with the content and to prevent malicious activity. Users also need to be able to find PWAs through search engines and easily share links through a URL and be able to install it easily.
Web apps that incorporate these criteria are progressive web apps. The main takeaways are that these apps improve usability and access. Users can access PWAs through Apple or Android stores and the apps themselves are easy to download and use.
PWAs are great ways to expand your service offering and gain new users. However, they’re not for every business case. Sometimes a native app makes more sense. It all depends on what goals you have for the project. To get a better understanding of those technologies, go to our blog post about choosing a tech stack and start your project right. If you want your app to increase its reach, consider creating a PWA. If your audience cares about preserving battery life or accessing the hardware of their devices, such as NFC, Bluetooth or a camera, you may want a native app.
In PWAs a service worker is a script that works independently in the browser’s background. These act a bit like proxies, but on the user side. Service workers allow you to manage push notifications, track network traffic and create so-called offline first web apps. Their ability to communicate with the server when it’s available, cache data and serve it later makes these components a vital part of a PWA.
A PWA’s web app manifest serves information about a web application in a JSON text file. PWA manifests include its name, author, icons, version, description, and a list of necessary resources. Web manifests enable users to install a PWA on any device and for the app to remain current and useful regardless of operating system or screen.
Application shells are a way to design app architecture so that your PWA loads on any device. This is similar to how native apps work, but it ensures a progressive web app loads quickly and reliably. The shell is a bare-bones HTML, CSS and JavaScript that loads and gets cached on a user’s device to quickly load the user interface. This speeds up load time by only loading necessary content, while saving what was already loaded.
import React from "react";
import ReactDom from "react-dom";
import { App } from "./App";
ReactDom.render(<App />, document.getElementById("root"));
First of all, we need to install service worker. A service worker is a script that your browser runs in the background, separate from a web page.
We start by creating a special JavaScript file, let’s call it serviceWorker.js. Inside it, we can use a special API provided by service worker for example to create cache strategies, but it’s out of scope today! What we will do, is to create the simplest cache strategy to test if our worker works. Just copy&paste this code inside.
import { precacheAndRoute } from "workbox-precaching";
console.log("Hello from Service Worker!");
precacheAndRoute(self.__WB_MANIFEST);
When we have our file ready, we need to tell the browser to load it into our page. We can do it in our main script like this:
window.onload = () => {
if ("serviceWorker" in navigator) {
navigator.serviceWorker.register("./serviceWorker.js");
}
};
What we do here is we wait until the page is loaded, and when it does, we check if the browser supports service workers. If you’re not sure your browser does it check this website.
In order to make our life easier, on top of what we have right now, we decided that it will be a good call to add Workbox to our example.
Workbox is a set of libraries to help you write and manage service workers and caching with the CacheStorage API. Service workers and the Cache Storage API, when used together, let you control how assets (HTML, CSS, JS, images, etc…) used on your site are requested from the network or cache, even allowing you to return cached content when offline! With Workbox, you can quickly set up and manage both, and more, with production-ready code.
We just need to add this code snip to our webpack config, to make it work.
plugins: [
...,
new WorkboxPlugin.InjectManifest({
swSrc: './src/serviceWorker.js',
swDest: 'serviceWorker.js',
}),
],
Now we should be able to see Service worker being loaded into the webpage.
Last step here is to create and install manifest.json
file, to turn our application into real PWA!
First of all, we start by creating manifest.json
file in src/
folder.
Next up, we need to fill the required fields in the manifest, in order to create the proper one. Here is a list of all available options and example config.
{
"name": "Applandeo app",
"short_name": "pwa app",
"theme_color": "#258fe3",
"background_color": "#ededed",
"display": "standalone",
"start_url": "/",
"icons": [
{
"src": "pwa512.png",
"type": "image/png",
"sizes": "512x512"
},
{
"src": "pwa192.png",
"type": "image/png",
"sizes": "192x192",
"purpose": "any maskable"
}
]
}
Once we have our manifest file ready, we just need to add one liner to HTML file in order to load it:
<link rel="manifest" href="manifest.json" />
The last thing we can do is to verify that our example is correct. To do so, we use the Lighthouse tool that we can find in the Chrome browser.
Now we generate report to see if have valid application.
And everything works as expected.
Progressive web apps are great tools to increase the usability of your app and to extend your user base. Since these apps operate independently of browser type or device, you can attract people to the app who would otherwise bounce. It’s also a great way to bypass app stores and allow users to quickly install and start using your web app. Many popular services have increased user engagement as well as the number of cerversions users complete on the PWA. These types of web apps can also greatly reduce development time and the cost to bring an app to the market. But this should not be your only calculation.
These apps are straightforward to build, but compe with a few drawbacks, especially when it comes to mobile battery life and access to device features, such as camera access, NFC and Bluetooth. If these functions are important to your business goals or to your end users, consider developing a native app. However, if this doesn’t affect your service’s usability, a progressive web app can be a solid alternative.
As with anything in web application development, listen to and observe your end-users to decide what’s most useful to them. If speed and offline usability is important, build a PWA, if not, stick to native applications.
Are you looking for a tech partner? Searching for a new job? Or do you simply have any feedback that you'd like to share with our team? Whatever brings you to us, we'll do our best to help you. Don't hesitate and drop us a message!
Drop a message